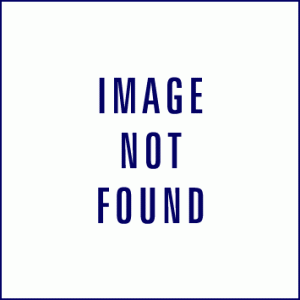
Missing or broken images can be a pain, especially since in some cases it's next to impossible to programatically figure out there's something wrong until you've reached the templating (view) layer. Here's a jQuery solution for those cases!
My scenario - a client's site has a "premium" feed that includes images. These images are scans created by the feed generating company. However, there are situations where the images they provide (which are not hosted at my clients website) are corrupted (as in, half the image is correct, the other is green with pixels scattered).
You could of course set up a server-side checking method, which requests the image, runs a few checks ( getimagesize() gets my vote in those situations ), and only displays the correct images. However this is bound to be slow, since you add a lot of latency (you need to wait for the other server to reply, then get and process the image before you start serving a page).
Long story short, here's what you need to do to hide the image ( or replace it! ) using jQuery ( any version ): you bind the error event of the all "interesting" images (the ones you think might be problematic, that is), and the act upon them. The following snippet will hide the image container (in order to hide accompanying text) -
$(document).ready(function() { /* Hide broken titles */ $(".image-container img").error(function() { $(this).parents('.image-container').hide(); }); });
Or you could simply use a default image:
$(document).ready(function() { /* Hide broken titles */ $(".image-container img").error(function() { $(this).attrib('src','/path/to/default/image.jpg'); }); });
In either case, you don't weigh down on your page generation time, and you control what your visitor sees. Do not that this is not a "nice" method of dealing with the problem, since some people with slower connections will see the image remnant before the snippet is executed - the "proper" way to deal with this situation would be to initially hide all images, then display those that don't have errors. However, assuming that more often than not, your images are ok, and that you prefer a script running only if there are problems instead of a script running on every page load... this is good enough.
Hope some people will find this helpful!